Make a note of the procedure to build a node.js environment with express and webpack using Docker. (* Because I am a beginner, if there are any mistakes or misunderstandings, please let me know in the comments.)
First, type the following command in the terminal to create the directory and file.
mkdir myapp
cd myapp
touch Dockerfile docker-compose.yml package.json \
webpack.config.js
mkdir views public
Now, the structure of the myapp folder is as follows.
myapp
├── Dockerfile
├── docker-compose.yml
├── package.json
├── public
├── views
└── webpack.config.js
First, create package and json.
In package.json, it is also possible to fill in the required package for the application in advance.
It is possible to create it with npm init -y
, but this time I will explain how to write the package information in advance.
In my environment, the structure of package.json is as follows.
{
"name": "app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"bootstrap": "^4.5.3",
"ejs": "^3.1.5",
"express": "^4.17.1",
"jquery": "^3.5.1",
"js": "^0.1.0",
"popper.js": "^1.16.1",
"node-sass": "^5.0.0",
"postcss-loader": "^4.0.4",
"sass": "^1.29.0",
"sass-loader": "^10.0.5",
"style-loader": "^2.0.0",
"webpack": "^5.4.0",
"webpack-cli": "^4.2.0",
"css-loader": "^5.0.1",
"extract-text-webpack-plugin": "^4.0.0-beta.0"
},
"devDependencies": {
"autoprefixer": "^10.0.1",
"fibers": "^5.0.0"
}
}
here
npm install -D package#Save to devDependencies → Development environment
npm install -S package#Save to production environment
It should be noted that it will be done.
Next, create a Dockerfile. By using Dockerfile, you can create a development environment at high speed without any difference. The contents of the Dockerfile are described as follows.
#Specify the version of nodejs.
FROM node:12.0
#Install required packages in os(It is recommended to write it as a spell)
RUN apt-get update && apt-get install -y \
#Because non-root users use root privileges
sudo \
#Get tools from internet
wget \
#Use vim as an editor
vim
#Creating an application directory
RUN mkdir /app
#Use app directory as development directory
WORKDIR /app
#Package in container.json and packate-lock.Make sure that two of json are copied
COPY package*.json ./
# package.Install the package described in json.
RUN npm i
#installed node_Copy files such as module to the container side.
COPY . .
CMD ["node","app.js"]
You can launch several docker containers at the same time by using docker-compose.yml. Since we don't use the database this time, we only launch the node.js container. Describe as under docker-compose.yml.
# docker-Specify the compose version
version: "3"
services:
app:
#Build a Dockerfile in the same directory
build: .
#Give the container a name(Any)
container_name: nodesam
#You can keep the container running.
tty: true
#Mount the files in the directory to the app directory in the container
volumes:
- .:/app
#Mount port 1000 on the container side to port 8080 on the host side.
ports:
- "8080:8080"
By creating webpack.config.js
npx webpack
You can compile files such as scss and typescript into one js file by typing the command. You can check the outline by looking at webpack official website, so please check it. The actual file structure is Compile css and scss using webpack
const path = require('path');
module.exports = {
//mode is production/Described in development
// ""Note that it is surrounded by
mode: "development",
//Which file to read default=> ./src/index.js
entry: './public/index.js',
//Where to compile the file read by entry
output: {
path: path.resolve(__dirname, 'public'),
//sample to dist.Spit out with the file name js
filename: 'sample.js',
},
module: {
rules: [
//Read and compile Sass files
{
//Target files with extensions sass and scss
test: /\.s[ac]ss$/i,
//Loader name
use: [
//Function to output to link tag
"style-loader",
//Ability to bundle CSS
"css-loader",
// sass2css
"sass-loader",
],
},
{
//The extension of the target file
test: /\.(gif|png|jpg|eot|wof|woff|ttf|svg)$/,
//Import images as Base64
type: "asset/inline",
},
],
},
// ES5(IE11 etc.)Designation for (required for webpack 5 and above)
target: ["web", "es5"],
};
Run the following command to create index.ejs and style.scss.
touch public/style.scss views/index.ejs
touch app.js
For the time being, configure the contents of index.ejs to the minimum.
index.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Hello world</h1>
<script src="./sample.js"></script>
</body>
</html>
Next, write style.scss as follows.
body{
background: red;
}
h1{
color: aqua;
}
Finally, create app.js so that you can check the ejs file via http communication. The configuration is as follows.
const express = require('express');
const path = require('path');
const app = express();
const ejs = require("ejs");
// host:port 0.0.0.0:8080
//0 on host.0.0.Map port 0 to port 8080.
const PORT = 8080;
const HOST = '0.0.0.0';
//Based on public file → This allows you to read css and javascript in public
app.use(express.static("public"));
//Make ejs readable
app.set('ejs',ejs.renderFile)
app.get('/', (req, res) => {
res.render("index.ejs")
});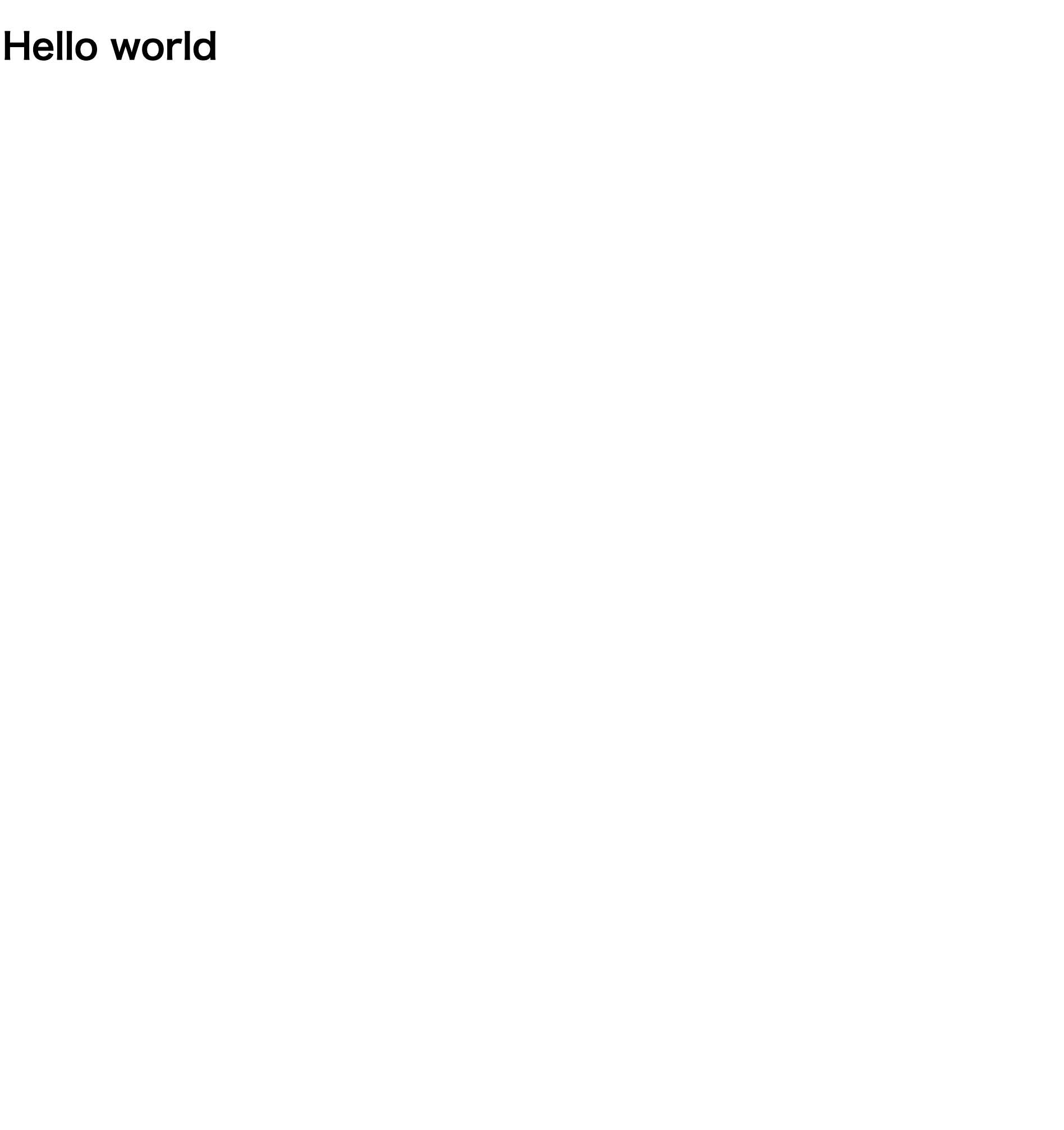
app.listen(PORT, HOST);
console.log(`Running on http://${HOST}:${PORT}`);
Actually start the server with docker-compose. Command the commands in the following order.
docker-compose build
docker-compose up
docker-compose build: Create a container based on docker-compose.yml docker-compose up: Launch the container created by docker-compose build
After the following command,
nodesam | Running on http://0.0.0.0:8080
Is displayed, so when you actually access http://0.0.0.0:8080
, you can see a simple site where the background is white and Hello World is output as shown below.
The style.scss created earlier does not work because it is not read in index.ejs. So compile to style.scss → sample.js with webpack.
First, create a js file to be used for compilation in public.
touch public/index.js
This time, only style.scss is compiled, so index.js is as follows.
index.js
import "./style.scss"
Use the following command to compile. First, move inside the container with the following command,
host terminal
docker exec -it nodesam bash
Terminal in docker container
npx webpack
This will compile style.scss-> style.js-> sample.js and spit out a file called sample.js in the public directory.
Now, when you access http://0.0.0.0:8080
again, you can see that the contents of style.scss are reflected as shown below. (Color disgusting)
That's all for this time.
The code created this time is posted on the following github, so for reference.
・ How to write Dockerfile Description of docker-compose.yml ・ How to use webpack
Recommended Posts