This article assumes that you have ev3dev installed on your EV3 and have an SSH connection. If you have not built the environment, please refer to this article.
mindstorm-Let's control EV3 with Linux! Install ev3dev OS and SSH connection
I will introduce how to display a bmp image on the LCD screen using ev3dev-lang-python of the python ev3dev library. The method described in the official documentation didn't work, so I hope you find this article helpful.
A bmp image of a size suitable for LCD display was provided on the library homepage.
Download the zip file, unzip it, and save it to EV3.
BMP image collection
Let's display a bmp image on the LCD.
The official documentation (https://media.readthedocs.org/pdf/python-ev3dev/latest/python-ev3dev.pdf) describes the image method.
image Returns a handle to PIL.Image class that is backing the screen. This can be accessed for blitting images to the screen. Example:
screen.image.paste(picture, (0, 0))
However, when I try to execute it, it seems that the image method is not defined and an error occurs.
robot@ev3dev:~$ python
Python 2.7.9 (default, Aug 13 2016, 17:33:18)
[GCC 4.9.2] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>>from PIL import Image, ImageDraw, ImageFont, ImageOps
>>>import ev3dev.auto as ev3
>>> screen = ev3.Screen()
>>>logo = Image.open('test.bmp')
>>> screen.image.paste(logo,(0,0))
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'Screen' object has no attribute 'image'
It worked fine with the following program.
bmp_lcd.py
mport ev3dev.auto as ev3
from PIL import Image, ImageDraw, ImageFont, ImageOps
screen = ev3.Screen()
logo = Image.open('EV3_BMPs/Awake.bmp')
#screen.draw.bitmap((0, 0), logo.convert("L")) #The color is reversed
screen.draw.bitmap((0, 0), ImageOps.invert(logo.convert("L"))) #success
screen.update()
logo.convert("L")Image with 8-Converting to monochrome of bit pixels.
However, in this state, there is a decrease in black and white being inverted when the LCD is displayed, so ```ImageOps.invert ()` `` is used to restore it.
It was displayed like this.
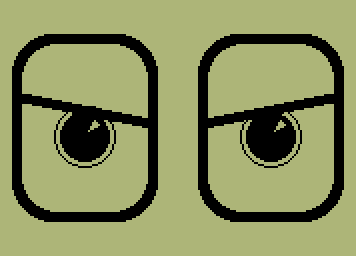
## reference
>
[https://sites.google.com/site/ev3python/learn_ev3_python/screen/bmp-image-collection](https://sites.google.com/site/ev3python/learn_ev3_python/screen/bmp-image-collection)
>
[http://pillow.readthedocs.io/en/3.3.x/handbook/concepts.html#modes](http://pillow.readthedocs.io/en/3.3.x/handbook/concepts.html#modes)
>
[[Ev3dev] Create a program to capture LCD (screen) using python](http://qiita.com/murotani/items/8f301c57d3e5741ea8f7)
Recommended Posts