brew update
brew install -v cmake
brew tap homebrew/science
brew install opencv
export PYTHONPATH="/usr/local/lib/python2.7/site-packages/:$PYTHONPATH"
Take an image of Messi and save it as "messi.jpeg "
#!/usr/bin/env python
# -*- coding: utf-8 -*-
import numpy as np
import cv2
#Load the original image
img = cv2.imread('messi.jpeg',cv2.IMREAD_UNCHANGED)
#Show in window
cv2.imshow("result", img)
#Export
cv2.imwrite('img.jpg', img)
#End processing
cv2.waitKey(0)
cv2.destroyAllWindows()
If it doesn't finish, press control + z to kill it
gray_img = cv2.imread('messi.jpeg', cv2.IMREAD_GRAYSCALE)
cv2.imshow("result", gray_img)
canny_img = cv2.Canny(gray_img, 50, 110)
cv2.imshow("result", canny_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
#Change the strength of the contour
cv2.Canny()Is a function that processes by the Canny method and sets two thresholds. The higher the number, the less contours will be written.
Click here for details http://postd.cc/image-processing-101/
canny_img = cv2.Canny(gray_img, 200, 400)
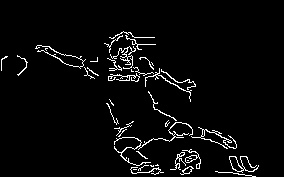
#Detect the ball
If the object of interest is a standard form such as a line or circle, it can be detected using the Hough transform.
circles = cv2.HoughCircles(canny_img, cv2.cv.CV_HOUGH_GRADIENT, dp=2, minDist=50, param1=20, param2=30, minRadius=5, maxRadius=20 ) print circles import matplotlib.pyplot as plt plt.imshow(canny_img) plt.show()
Parameters
dp ・ ・ ・ Increase if the resolution of the original image is reduced and detected during processing. For example, if it is 1, it will be processed with the same image quality, and if it is 2, it will be reduced to 1/2. minDist ・ ・ ・ Minimum distance between circles detected param1 ・ ・ ・ It seems that "the higher of the two thresholds used in Canny's edge detector". The lower it is, the more edges are detected. param2 ・ ・ ・ Threshold value at the time of center detection calculation. The lower it is, the more non-circular it is detected. minRadius ・ ・ ・ Minimum radius maxRadius ・ ・ ・ Maximum radius
Then, 5 candidates came out[x,y,radius]
[[[ 125. 51. 16.27882004] [ 193. 161. 5.83095169] [ 131. 155. 8.24621105] [ 71. 73. 18.02775574] [ 161. 91. 17.80449295]]]
Plot the circle on the original image
cups_circles = np.copy(img)
if circles is not None and len(circles) > 0: circles = circles[0] for (x, y, r) in circles: x, y, r = int(x), int(y), int(r) cv2.circle(cups_circles, (x, y), r, (255, 255, 0), 4) plt.imshow(cv2.cvtColor(cups_circles, cv2.COLOR_BGR2RGB)) plt.show()
print('number of circles detected: %d' % len(circles[0]))
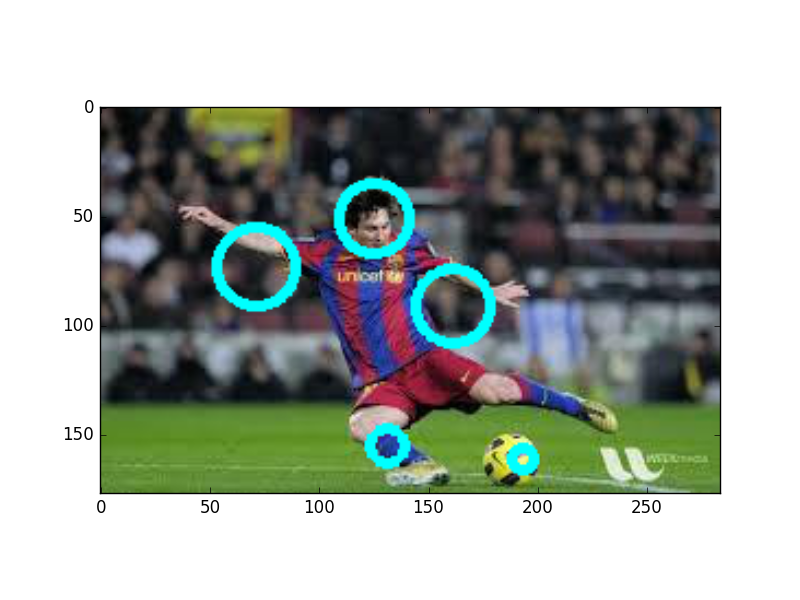
Hmm ... it's correct, but something strange is also counted
By adjusting the parameters etc., it was possible to make only the face and the ball
(I felt like I should stop grayscale)
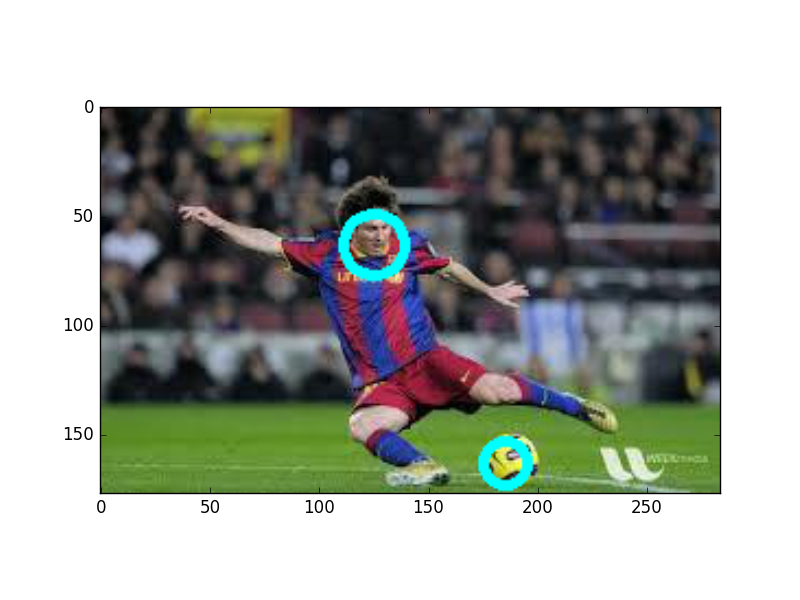
!/usr/bin/env python -- coding: utf-8 -- import numpy as np import cv2 import matplotlib.pyplot as plt
img = cv2.imread('messi5.jpeg',cv2.IMREAD_UNCHANGED) canny_img = cv2.Canny(img, 230, 500)
find hough circles circles = cv2.HoughCircles(canny_img, cv2.cv.CV_HOUGH_GRADIENT, dp=2, minDist=100, param1=25, param2=30, minRadius=5, maxRadius=20 ) print circles
cups_circles = np.copy(img)
if circles are detected, draw them if circles is not None and len(circles) > 0: # note: cv2.HoughCircles returns circles nested in an array. # the OpenCV documentation does not explain this return value format circles = circles[0] for (x, y, r) in circles: x, y, r = int(x), int(y), int(r) cv2.circle(cups_circles, (x, y), r, (255, 255, 0), 4) plt.imshow(cv2.cvtColor(cups_circles, cv2.COLOR_BGR2RGB)) plt.show()
cv2.waitKey(0) cv2.destroyAllWindows()
How. It looks more beautiful when you apply the color fista
http://stackoverflow.com/questions/22870948/how-can-i-pythonically-us-opencv-to-find-a-a-basketball-in-an-image
http://www.pyimagesearch.com/2015/09/14/ball-tracking-with-opencv/
If you make it into an application, it will be helpful
http://www.melt.kyutech.ac.jp/2015/onoue.pdf#search='opencv+ball+%E7%B2%BE%E5%BA%A6'
How can you make a chasing camera with Raspberry Pi!
https://www.youtube.com/watch?v=58xxn6d_bUg
Next, let's try a video tutorial
http://labs.eecs.tottori-u.ac.jp/sd/Member/oyamada/OpenCV/html/py_tutorials/py_gui/py_video_display/py_video_display.html
Recommended Posts