I wanted to start studying python, so I created a bot using Slackbot as a starting point. Added a simple conversation (reply to a specific word) function and a function that tells the weather / delay information. A memo at that time.
Install Python. Anaconda is convenient because it builds a Python environment. So, this time, I will introduce how to build an environment using Anaconda.
Press the play button and click Open Terminal
You can start the command prompt in the created environment
Create an account for your bot in Slack. Please see Slack Home Page for how to create Slack for your team.
While logged in as an authorized user, click here (https://my.slack.com/services/new/bot).
The Bot account creation screen opens.
Enter your preferred username and click Add bot integration.
You will need a part called API Token, so make a note of it.
Customize Bot appropriately.
Install Slackbot. You can do this by entering the following code at the command prompt in the environment created by Anaconda.
pip install slackbot
Create the following directory structure
Enter the following code in run.py
from slackbot.bot import Bot
def main():
bot = Bot()
bot.run()
if __name__ == "__main__":
print('start slackbot')
main()
Specify the token of the bot account.
Enter the following code in slackbot_settings.py
API_TOKEN = "API Token that I wrote down earlier"
#Response string when a message addressed to this bot does not apply to any response
DEFAULT_REPLY = "What are you talking about"
PLUGINS = [
'plugins',
]
1. After saving the code, display the command prompt again.
Change to the directory where run.py exists and execute the following command.
```python run.py```
1. Talk to the account you created.
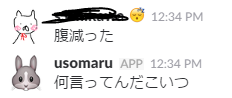
The bot "What are you talking about?" Is completed for all words.
# Let's have more conversation
## Respond to a specific word
You can add functionality by editing `my_mention.py` in the directory you just created.
Let's write the following code as an example.
#### **`my_mention.py`**
```python
from slackbot.bot import respond_to # @botname:Decoder that reacts with
from slackbot.bot import default_reply #Decoder that reacts when there is no corresponding response
@respond_to('Lie') #Words I want you to react to
def mention_func(message):
message.reply('The lie is a fake.') #Mention
Let's start it.
He responded to a specific word.
A brief explanation of the code.
First, about the plugins directory.
_Init_.py
created in the plugins directory
This was created because the directory to be loaded as a Slackbot plugin must be a Python package.
The contents are empty because it is only recognized as a package.
Then my_mention.py
Mainly write the code required for the function you want to add here.
By writing the words you want to respond to the respond_to
decorator argument, you will be registered to respond to mentions to the bot.
Since the mention
part of mention_func
is the faction name,
If you want to add a mention function to other words, change the name here.
Finally slackbot_settings.py
The code part below written here
slackbot_settings.py
PLUGINS = [
'plugins',
]
This is described so that the plugin can be loaded.
Therefore, any directory name can be entered in the plubins
part.
Use the Weather Information API (http://weather.livedoor.com/weather_hacks/webservice) to get today's weather information. This site returns data in json format, so Get the information you need from here.
This time, I will introduce today's weather and how to display the icon according to the weather.
Add the following code to my_mention.py
to add functionality.
my_mention.py
@respond_to('today's weather')
def weather(message):
import urllib
import json
url = 'http://weather.livedoor.com/forecast/webservice/json/v1?city='
# '130010'Then it will get information about Tokyo
#If you change this, you can get the weather information of any area.
city_id = '130010'
html = urllib.request.urlopen(url + city_id)
jsonfile = json.loads(html.read().decode('utf-8'))
title = jsonfile['title']
telop = jsonfile['forecasts'][0]['telop']
#If the telop is sunny, the icon of sunny slack or the case
telop_icon = ''
if telop.find('snow') > -1:
telop_icon = ':showman:'
elif telop.find('Thunder') > -1:
telop_icon = ':thinder_cloud_and_rain:'
elif telop.find('Fine') > -1:
if telop.find('Cloudy') > -1:
telop_icon = ':partly_sunny:'
elif telop.find('rain') > -1:
telop_icon = ':partly_sunny_rain:'
else:
telop_icon = ':sunny:'
elif telop.find('rain') > -1:
telop_icon = ':umbrella:'
elif telop.find('Cloudy') > -1:
telop_icon = ':cloud:'
else:
telop_icon = ':fire:'
text = title + '\n' + 'today's weather' + telop + telop_icon
message.send(text)
Let's hear the weather today.
Briefly explain the code again. First, the title and telop parts. Here, referring to the property name of the weather forecast API linked above, You can get the information attached to the property name by writing the property name you want in ['']. However, for the forecasts property obtained by telop, the data is inserted in the array format. The 0th in the array is today's weather, the 1st is tomorrow's weather, and the 2nd is the day after tomorrow's weather. This time I want to get today's weather, so I'm specifying the 0th in the array.
Next is the part that is branched by the if statement, Here, the slack icon to be displayed is branched based on the weather word acquired by telop.
Finally, the text part connects the words to be displayed on the bot. You can even get the highest temperature, so it's worth customizing in the future.
Finally, get the train delay information. Here Delay information Use JSON and use the same method as when the weather forecast was obtained.
Since the function is added again, add the following code to my_mention.py
.
my_mention.py
@respond_to('Are you late for the train?')
def train(message):
import urllib
import json
url = 'https://rti-giken.jp/fhc/api/train_tetsudo/delay.json'
html = urllib.request.urlopen(url)
jsonfile = json.loads(html.read().decode('utf-8'))
for json in jsonfile:
name = json['name']
company = json['company']
text = company + name + 'Is delayed ♪'
message.send(text)
I will display it.
I haven't customized it to display delay information by region yet, so Delay information nationwide will come out.
In the future, we plan to create a bot that will customize delay information for each region and introduce recommended restaurants.
-Create a Slack bot with Python's slackbot library -Making Slackbot with Python (1) -Making Slackbot with Python (2) -How to make a dialogue system dedicated to beginners -I created a Slack Bot using Python
-Slackbot memorandum (2) ~ bot using docomo chat dialogue API ~
Recommended Posts