A person who develops web systems using Django all the time. I recently started studying Flask because I wanted to make a simple app in my spare time. It's not good to pack too many settings in one file, so I've put together a directory structure based on MVC.
flask_app
├ common
│ ├ libs
│ └ models ---model
│ ├ user.py
│ └ ・ ・ ・
├ config ---Settings folder
│ ├ base_setting.py ---Common settings for each environment
│ ├ local_setting.py ---Settings for local development environment
│ └ production_setting.py ---Production environment settings
├ controllers ---controller
│ ├ index.py
│ └ ・ ・ ・
├ interceptors
│ ├ auth.py ---Authentication system processing
│ └ error_handler.py ---Error handling
├ static ---Static file location
├ templates ---template
│ ├ common
│ │ └ layout.html
│ └ index.html
├ application.py ---Define what is used by multiple files (Flask instance, DB, environment variables, etc.)
├ manager.py ---Application execution script (application entrance)
├ requirements.py ---Library list
└ www.py ---routing
I will explain in order.
app.config.from_pyfile("config/base_setting.py")First read the common settings with. The settings that change depending on the environment (development / production) are the environment variables ops set in advance._Changed to read different config file depending on different value of config.
#### **`flask_app/application.py`**
```flask
from flask import Flask
from flask_script import Manager
from flask_sqlalchemy import SQLAlchemy
import os
app = Flask(__name__)
manager = Manager(app)
app.config.from_pyfile("config/base_setting.py")
# linux export ops_config=local|production
# windows set ops_config=local|production
if "ops_config" in os.environ:
app.config.from_pyfile("config/%s_setting.py" % (os.environ['ops_config']))
db = SQLAlchemy(app)
The contents of controllers are divided by function and integrated using blueprint at www.py.
flask_app/www.py
from interceptors.errorHandler import *
from interceptors.Auth import *
from application import app
from controllers.index import index_page
from flask_debugtoolbar import DebugToolbarExtension
toolbar = DebugToolbarExtension(app)
app.register_blueprint(index_page, url_prefix="/")
Define the model in models. Here, a test model is defined in user.py.
flask_app/common/models/user.py
from application import db
class User(db.Model):
__tablename__ = 'user'
id = db.Column(db.Integer, primary_key=True, autoincrement=True)
name = db.Column(db.String(20), nullable=False)
Define the actual processing in controllers.
flask_app/controllers/index.py
from flask import Blueprint, render_template
from common.models.user import User
index_page = Blueprint("index_page", __name__)
@index_page.route("/")
def index():
name = "hello"
context = {"name": name}
context["user"] = {"nickname": "xxxx",
"id": "xxxxx", "home_page": "http://www.xxx"}
context["num_list"] = [1, 2, 3, 4, 5]
result = User.query.all()
context['result'] = result
return render_template("index.html", **context)
Register the commands runserver and create_all with the manager's add_commend method.
flask_app/manager.py
from application import app, db, manager
from flask_script import Server, Command
from www import *
# web server
manager.add_command("runserver", Server(host = "0.0.0.0", use_debugger=True, use_reloader=True))
# create tables
@Command
def create_all():
from common.models.user import User
db.create_all()
manager.add_command("create_all", create_all)
def main():
manager.run()
if __name__ == "__main__":
# app.run( host = "0.0.0.0" )
try:
import sys
sys.exit( main() )
except Exception as e:
import traceback
traceback.print_exc()
`pip install -r requirements.py`
to install the required modules.`flask_app / local_setting.py`
, set the user, password, host and port of db according to your environment.
python manager.py runserver
andhttp://localhost:Access 5000 and succeed when the next screen appears!
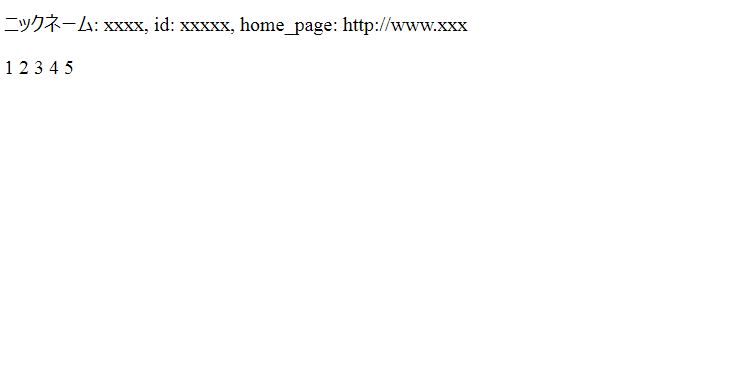
## Source
I uploaded the source to gitHub, so if you have time, please download it (https://github.com/shixujapan/flask_app) and play with it.
## What to do next
* Create a simple CRUD app using Flask
* Consider cooperation with Vue.js
Recommended Posts