There are various buttons in kivy. From the ones that can be used normally to the ones that are a little unclear. So, this time, I would like to pick up and introduce the ones that are especially likely to be used. I won't go into the details. It's not like making something using kivy's ui and introducing it like before. This article only shows examples of simple usage. The source is an official reference sale. Please refer to the reference for detailed usage!
I don't think it's necessary to introduce it, but it's an ordinary button.
button_sample.py
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.button import Button
from kivy.uix.label import Label
from kivy.uix.togglebutton import ToggleButton
from kivy.uix.behaviors import ToggleButtonBehavior
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
#Label definition
self.label = Label(text="OFF")
self.add_widget(self.label)
#Button definition, processing when pressed and spoken
self.button = Button(on_press=self.press, on_release=self.release, text="Press!")
self.add_widget(self.button)
#When pressed
def press(self, btn):
self.label.text = "This is Button!"
#When talking
def release(self, btn):
self.label.text = "OFF"
class Sample(App):
def build(self):
return Test()
Sample().run()
It is a button that you can check the ON status and OFF status when you press it.
Whether or not it is pressed can be determined by the variables `down``` and
normal``` of the toggle button ``` state```. Also, by setting ``
group```, it seems that you can use it like a radio button.
It seems that it is possible to give the image a toggle box-like behavior. (Unverified) https://kivy.org/doc/stable/api-kivy.uix.behaviors.togglebutton.html#kivy.uix.behaviors.togglebutton.ToggleButtonBehavior
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.label import Label
from kivy.uix.togglebutton import ToggleButton
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
#Label definition,
self.label = Label(text="OFF")
self.add_widget(self.label)
#Toggle button definition
self.toggle = ToggleButton(on_press=self.t_press, text="Press!")
self.add_widget(self.toggle)
#Paste the toggle button that is treated as a check box into a separately prepared box layout
self.toggle_box = BoxLayout()
self.t1 = ToggleButton(group="g1", on_release=self.toggle_check, text="toggle 1")
self.t2 = ToggleButton(group="g1", on_release=self.toggle_check, text="toggle 2")
self.t3 = ToggleButton(group="g1", on_release=self.toggle_check, text="toggle 3")
self.toggle_box.add_widget(self.t1)
self.toggle_box.add_widget(self.t2)
self.toggle_box.add_widget(self.t3)
self.add_widget(self.toggle_box)
def t_press(self, btn):
if btn.state == "down":
self.label.text = "This is Toggle Button!"
if btn.state == "normal":
self.label.text = "OFF"
def toggle_check(self, btn):
self.label.text = btn.text
class Sample(App):
def build(self):
return Test()
Sample().run()
Radio buttons and checkboxes. It behaves like a toggle button.
group
If set to, it becomes a radio button, otherwise it becomes a check box. (You can check it by removing the comment part of the following source.)
Unlike the toggle button, you can check if it is pressed with True False from the variable active
.
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.label import Label
from kivy.uix.checkbox import CheckBox
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
self.orientation = "vertical"
#Label definition, paste on screen
self.label = Label(text="OFF")
self.add_widget(self.label)
#Paste the toggle button that is treated as a check box into a separately prepared box layout
self.checkbox_box = BoxLayout(orientation="horizontal")
#Define checkbox
self.t1 = CheckBox()
self.t2 = CheckBox()
self.t3 = CheckBox()
#Define radio buttons
# self.t1 = CheckBox(group="g1")
# self.t2 = CheckBox(group="g1")
# self.t3 = CheckBox(group="g1")
self.checkbox_box.add_widget(self.t1)
self.checkbox_box.add_widget(self.t2)
self.checkbox_box.add_widget(self.t3)
#Processing when the check box is pressed
self.t1.bind(active=self.on_checkbox_active)
self.t2.bind(active=self.on_checkbox_active)
self.t3.bind(active=self.on_checkbox_active)
self.add_widget(self.checkbox_box)
def on_checkbox_active(self, instance, value):
self.label.text = "Left : {}\n" \
"Center : {}\n" \
"Right : {}\n".format(self.t1.state, self.t2.state, self.t3.state)
class Sample(App):
def build(self):
return Test()
Sample().run()
The one introduced above was a button properly, but I think that the one introduced below recognizes that something is done using the button.
It is a combo box. Please note that the list cannot be opened unless you open a space above or below it.
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.button import Button
from kivy.uix.label import Label
from kivy.uix.dropdown import DropDown
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
self.label = Label(text="test")
self.add_widget(self.label)
self.dropdown = DropDown()
for index in range(10):
btn = Button(text='Value %d' % index, size_hint_y=None, height=44)
#Processing when the defined button is pressed
btn.bind(on_release=lambda btn: self.dropdown.select(btn.text))
#Put a button on the dropdown
self.dropdown.add_widget(btn)
self.mainbutton = Button(text="main", size_hint=(1, 0.1), pos_hint={"y": 0.9})
self.mainbutton.bind(on_release=self.dropdown.open)
self.add_widget(self.mainbutton)
self.dropdown.bind(on_select=self.press)
def press(self, instance, x):
self.mainbutton.text = x
self.label.text = "Press : {}".format(x)
class Sample(App):
def build(self):
return Test()
Sample().run()
If you press it, a drop-down list will appear. It's similar to a combo box, but I think it has a higher degree of freedom because you can add functions to the opened buttons. I couldn't use it well. .. ..
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.button import Button
from kivy.uix.label import Label
from kivy.uix.dropdown import DropDown
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
self.label = Label(text="test")
self.add_widget(self.label)
self.dropdown = DropDown()
for index in range(10):
btn = Button(text='Value %d' % index, size_hint_y=None, height=44)
#Processing when the defined button is pressed
btn.bind(on_release=lambda btn: self.dropdown.select(btn.text))
#Put a button on the dropdown
self.dropdown.add_widget(btn)
self.mainbutton = Button(text="main", size_hint=(1, 0.1), pos_hint={"y": 0.9})
self.mainbutton.bind(on_release=self.dropdown.open)
self.add_widget(self.mainbutton)
self.dropdown.bind(on_select=self.press)
def press(self, instance, x):
self.mainbutton.text = x
self.label.text = "Press : {}".format(x)
class Sample(App):
def build(self):
return Test()
Sample().run()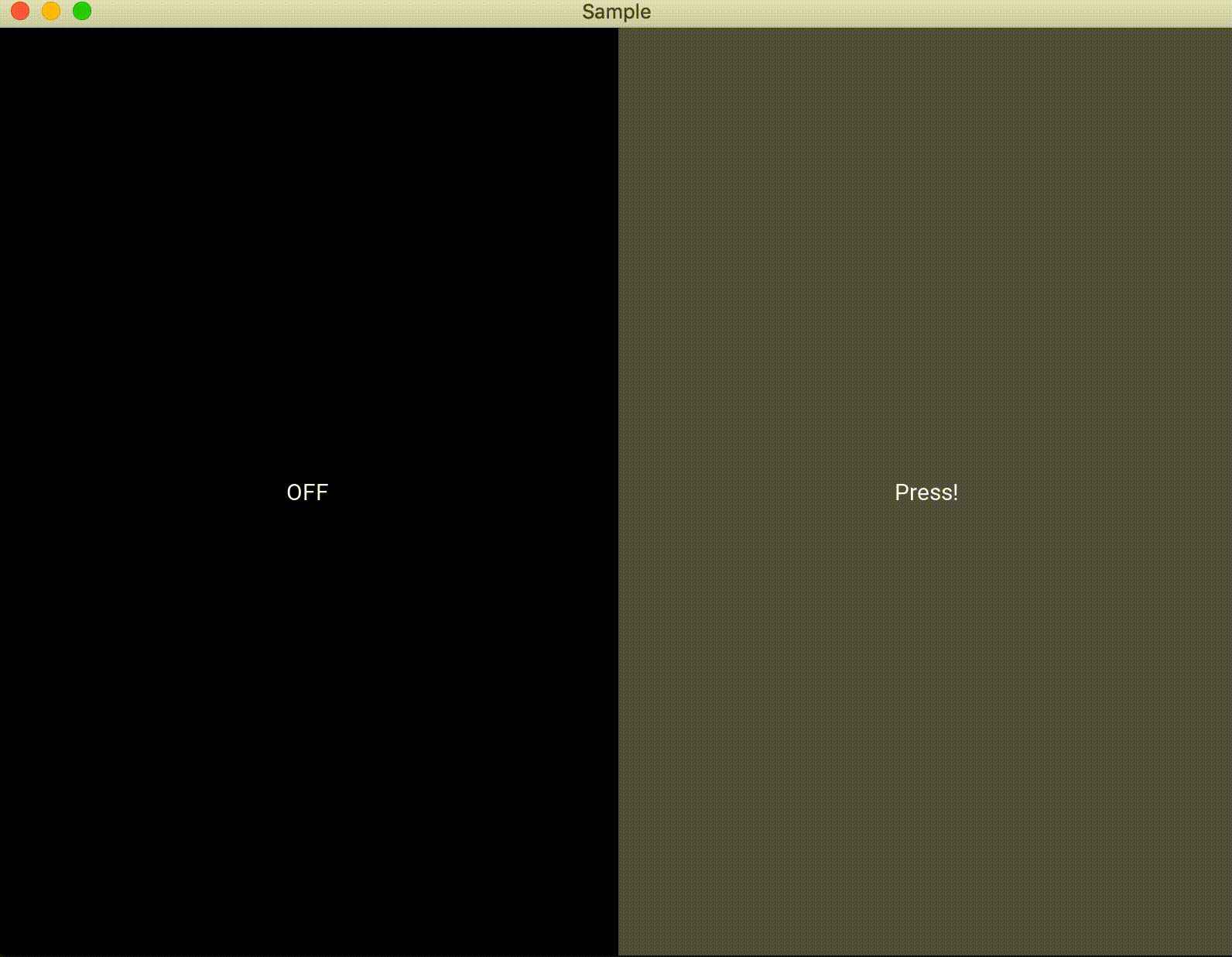
It is a switch. I have never used it. I think the behavior itself is almost the same as the checkbox. You can also hold the button and slide it, so I thought it was a little interesting w
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.label import Label
from kivy.uix.switch import Switch
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
self.label = Label(text="test")
self.add_widget(self.label)
self.switch = Switch()
self.switch.bind(active=self.switch_check)
self.add_widget(self.switch)
def switch_check(self, instance, value):
if value:
self.label.text = "ON"
else:
self.label.text = "OFF"
class Sample(App):
def build(self):
return Test()
Sample().run()
It is a list that comes out with Biro. I have never used this either.
If you want to put something in the list, put ids
when declaring AccordionImems
, which is a child element of Accordion
, or put an array. It may be possible if you hold it. (Unverified)
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.label import Label
from kivy.uix.accordion import Accordion, AccordionItem
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
self.label = Label(text="test")
self.add_widget(self.label)
self.accordion = Accordion(orientation='vertical')
#Define the contents of the Accordion
for x in range(5):
item = AccordionItem(title='Title %d' % x)
item.add_widget(Label(text='Very big content\n' * 10))
self.accordion.add_widget(item)
self.add_widget(self.accordion)
class Sample(App):
def build(self):
return Test()
Sample().run()
It is a button to switch tabs. Rather, I don't think this is a button anymore. This is a panel. I think this is a convenient class because you can easily switch screens without mounting screen fibers.
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.uix.label import Label
from kivy.uix.tabbedpanel import TabbedPanel, TabbedPanelItem
class Test(BoxLayout):
def __init__(self, **kwargs):
super(Test, self).__init__(**kwargs)
self.tabbedPanel = TabbedPanel(do_default_tab=False)
#Definition of the panel to be placed on the Tabbed Panel
self.panel1 = TabbedPanelItem(text="tab 1")
self.panel1.add_widget(Label(text="This is Panel 1"))
#Definition of the panel to be placed on the Tabbed Panel
self.panel2 = TabbedPanelItem(text="tab 2")
self.panel2.add_widget(Label(text="This is Panel 2"))
#Place on Tabbed Panel
self.tabbedPanel.add_widget(self.panel1)
self.tabbedPanel.add_widget(self.panel2)
#Put on App
self.add_widget(self.tabbedPanel)
class Sample(App):
def build(self):
return Test()
Sample().run()
It was a good opportunity to touch a button (?) That I had never touched before, even though it was an introduction. I briefly introduced the details without touching on them at all, but I felt that I would like to study a little more so that the button introduced this time can be used at another time.
Recommended Posts