[PYTHON] Move the Augmentaiton of Albumentations earnestly
What is Albumentations?
- https://github.com/albumentations-team/albumentations
- Python library for data extension for machine learning
- A wealth of features commonly used in Data augmentation
pip install albumentations
This article
Blur
Blur
- Blur with a randomly sized kernel
- blur_limit (int) – Maximum blurr kernel size. Default: (3, 7)

MotionBlur
- Apply motion blur (box filter) with random kernel size
- blur_limit (int) – Maximum blurr kernel size. Default: (3, 7).

GaussianBlur
- Apply Gaussian filter with random kernel size
- blur_limit (int) – Maximum kernel size to blur, must be odd. Default: (3, 7).

GlassBlur
- Adds glass noise (effect like frosted glass)
- sigma (float) – Standard deviation of the Gaussian kernel. Defalt: 0.7.
- max_delta (int) – Maximum distance between swapped pixels. . Defalt: 4.
- iterations (int) – Number of repeats. Default: (2).
- mode (str) – Computation mode. Fast or exact. Default: “fast”.

Noise, Compression
GaussNoise
- Add Gaussian noise
- var_limit ((float, float) or float) – Noise distribution. Default: (10.0, 50.0).
- mean (float) – Noise average. Default: 0

JpegCompression
- Apply Jpeg compression noise
- quality_lower (float) – The lower limit of quality.
- quality_upper (float) – Quality limit.

ImageCompression
- Apply Jpeg / WebP compression noise
- quality_lower (float) – The lower limit of quality.
- quality_upper (float) – Quality limit.
- compression_type (ImageCompressionType) – Compression type (JPEG / WEBP). should be ImageCompressionType.JPEG or ImageCompressionType.WEBP. Default: ImageCompressionType.JPEG

ISONoise
- Add camera sensor noise
- color_shift (float, float) – amount of hue change.
- intensity ((float, float) – Intensity of color / luminance noise.

MultiplicativeNoise
- Multiply by random number array
- multiplier (float or tuple of floats) – A range of numbers to multiply. Default: (0.9, 1.1).
- per_channel (bool) – False: Same for all channels, True: Use sample values for each channel. Default False.
- elementwise (bool) – False: Multiply all pixels in the image, True: Randomly sample and multiply pixel by pixel. Defaule: False.

Downscale
- Downscale and then upscale to reduce image quality
- scale_min (float) – The lower limit of the scale. Should be <1. Default: 0.25.
- scale_max (float) – The upper limit of the scale. Default: 0.25.

Simple geometric change system (Flip, Crop, Rotate, Scale, Transpose)
Flip
- Randomly flips horizontally, vertically, or both horizontally and vertically
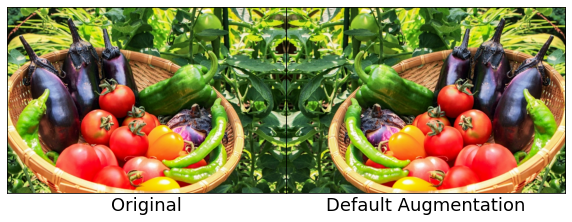
VerticalFlip
- Flip vertically around the x-axis
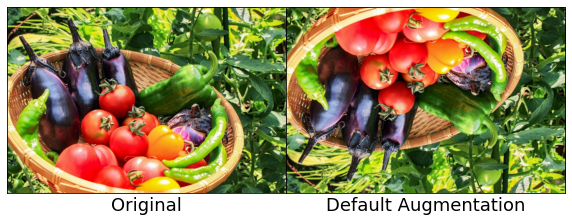
HorizontalFlip
- Flip horizontally around the y-axis
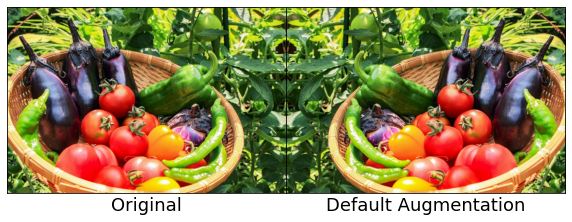
Crop
- Cut out the area
- x_min (int) – The minimum value of the upper left x coordinate.
- y_min (int) – The minimum value of the upper left y coordinate.
- x_max (int) – Maximum value of the lower right x coordinate.
- y_max (int) – Maximum value of the lower right y coordinate.
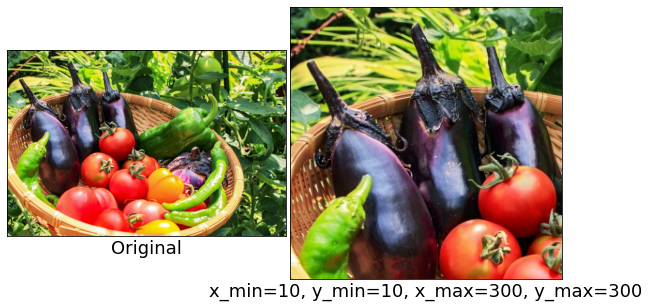
RandomCrop
- Randomly cut
- height (int) – The height to cut.
- width (int) – The width to cut.
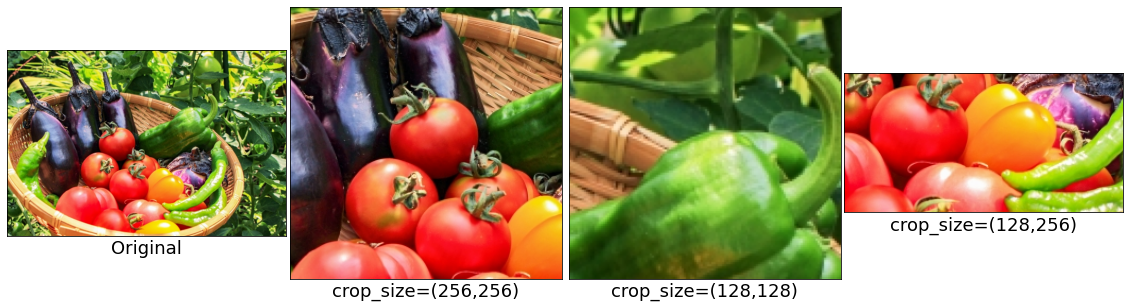
CenterCrop
- Crop the center part
- height (int) – The height to crop.
- width (int) – The width to crop.
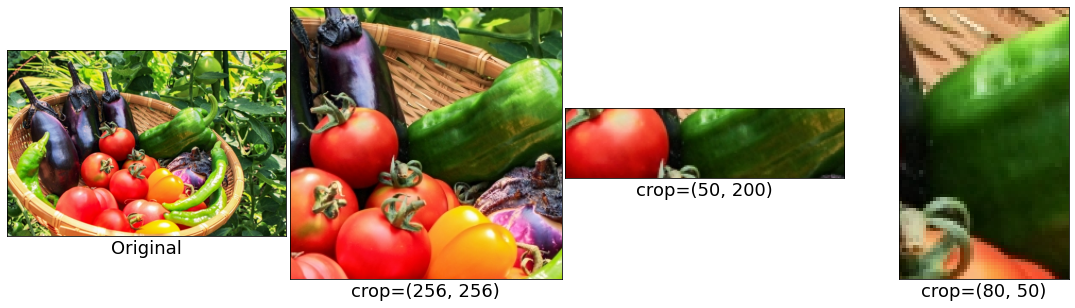
RandomSizedCrop
- Randomly cut and rescale to a specific size
- min_max_height ((int, int)) – Crop size range.
- height (int) – Height after resizing.
- width (int) – Width after resizing.
- w2h_ratio (float) – Crop aspect ratio. Default: 1.0.
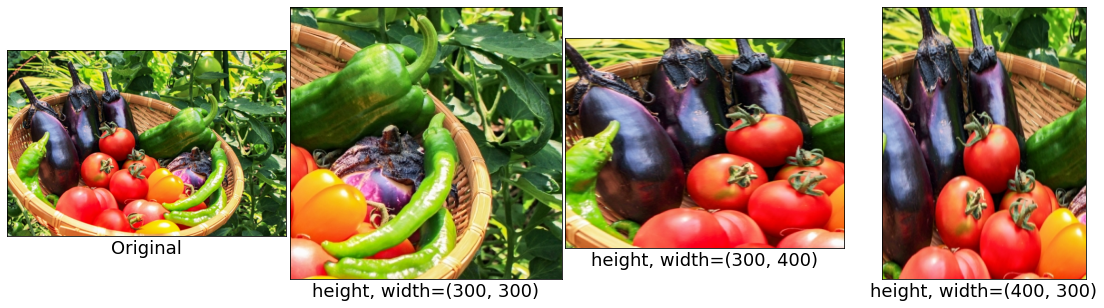
RandomResizedCrop
- Randomly cut and rescale to a specific size (Torchvision variant)
- height (int) – Height after resizing.
- width (int) – Width after resizing.
- scale ((float, float)) – The size range of the area to crop. Default: (0.08, 1.0).
- ratio ((float, float)) – The range of aspect ratios for the area to be cropped. Default: (0.75, 1.3333333333333333).
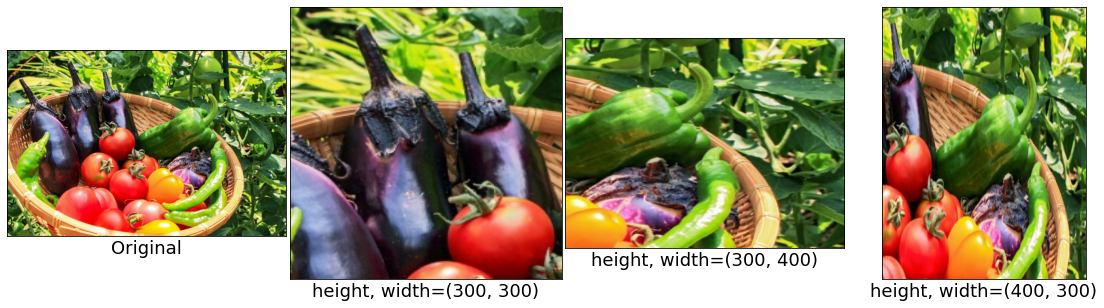
Rotate
- Rotate at random angles
- limit: Range of angles, (-limit, limit) for single numbers. Default: (-90, 90)

RandomScale
- Randomly change the image size
- scale_limit ((float, float) or float) – Scaling range (note that 0 is unchanged). Default: (0.9, 1.1).

- Since it is previewed in the same size, the original image size has changed although it is not visible.
RandomRotate90
- Randomly rotate in 90 ° increments
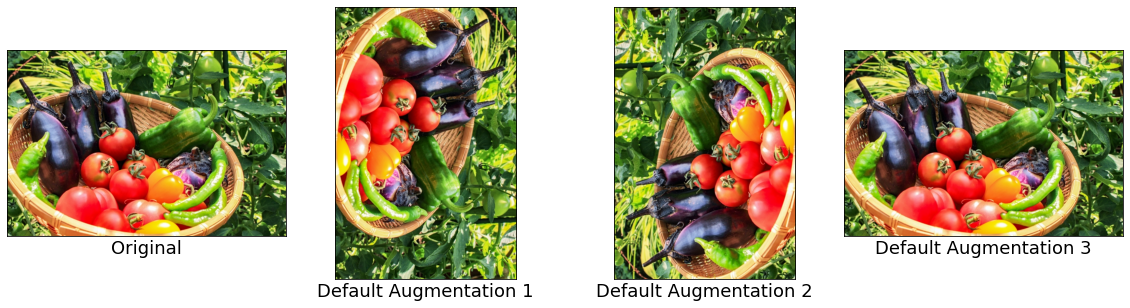
Transpose
- Transpose rows and columns
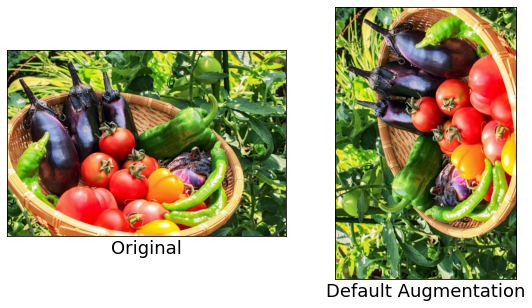
Advanced geometric transformation system (Affine, Distortion)
ShiftScaleRotate
- Randomly apply affine transformations (translation, scaling, rotation)
- shift_limit: The range of translation. Default: (-0.0625, 0.0625).
- scale_limit: Scale range (note that 0 is unchanged). Default: (-0.1, 0.1).
- rotate_limit: rotation range. The range of rotation. Default: (-45, 45).

OpticalDistortion
- Reproduce optical distortion
- distort_limit (float, (float, float)) – Range of distortion. Default: (-0.05, 0.05).
- shift_limit (float, (float, float))) – The range to shift. Default: (-0.05, 0.05).
- Not applicable to Bounding Box and Keypoints

GridDistortion
- Reproduce Grid distortion
- num_steps (int) – Specify the number of grid cells on each side. Default: 5.
- distort_limit (float, (float, float)) – Range of distortion. Default: (-0.03, 0.03).
- Not applicable to Bounding Box and Keypoints

ElasticTransform
- Elastic deformation
- alpha (float) – transformation parameters. Default: 1.
- sigma (float) – Gaussian filter parameters. Default: 50.
- alpha_affine (float) – Range of alpha_affine. Default: 50.
- Not applicable to Bounding Box and Keypoints

RandomGridShuffle
- Randomly shuffle cells in the grid
- grid ((int, int)) – The size of the grid that divides the image. Default: (3,3).
- Not applicable to Bounding Box and Keypoints

Color tinkering system
HueSaturationValue
- Randomly change hue, saturation, and brightness
- hue_shift_limit ((int, int) or int) – Hue range. Default: (-20, 20).
- sat_shift_limit ((int, int) or int) – Saturation range. Default: (-30, 30).
- val_shift_limit ((int, int) or int) – Brightness range. Default: (-20, 20).

RGBShift
- Randomly change the value of each RGB channel
- r_shift_limit ((int, int) or int) – Red channel range of change. Default: (-20, 20).
- g_shift_limit ((int, int) or int) – Green channel range of change. Default: (-20, 20).
- b_shift_limit ((int, int) or int) – Blue channel range of change. Default: (-20, 20).

ChannelShuffle
- Randomly sort RGB channels

ChannelDropout
- Randomly drop channels
- channel_drop_range (int, int) – The range of channels to drop.
- fill_value (int, float) – Pixel value to fill the dropped channel instead.

Posterize
- Reduce the number of bits in each color channel
- num_bits ((int, int) – range of bits. Default: 4.

ToGray
- Convert RGB image to grayscale
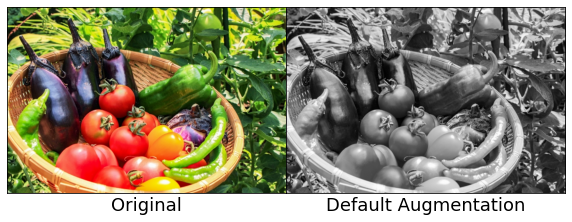
ToSepia
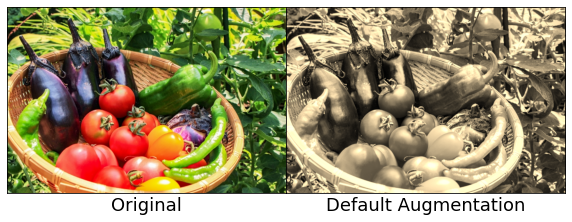
Brightness and contrast tinkering system
InvertImg
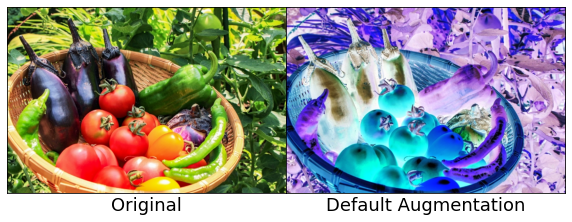
Normalize
- Divide the pixel value by 255 → subtract the average value for each channel → divide by the standard deviation for each channel
- ** Mean and standard deviation are just parameters and are not implicitly calculated internally **
- mean (float, list of float) – Mean. Dafault: (0.485, 0.456, 0.406).
- std (float, list of float) – Standard deviation. Dafault: (0.229, 0.224, 0.225).
- max_pixel_value (float) – Maximum pixel value. Dafault: 255.0

RandomGamma
- Randomly apply gamma conversion
- gamma_limit (float or (float, float)) – The upper limit of gamma. Default: (80, 120).

RandomBrightness
- Randomly change the brightness
- limit ((float, float) or float) – Range of change in brightness. Default: (-0.2, 0.2).

RandomContrast
- Randomly change the contrast
- limit ((float, float) or float) – Range of change in contrast. Default: (-0.2, 0.2).

RandomBrightnessContrast
- Randomly change brightness and contrast
- brightness_limit ((float, float) or float) – The range of change in brightness. Default: (-0.2, 0.2).
- contrast_limit ((float, float) or float) – Contrast range of change. Default: (-0.2, 0.2).
- brightness_by_max (Boolean) – How to adjust the contrast. Default: True.

CLAHE
- Contrast limit adaptive histogram equalization
- clip_limit (float or (float, float)) – The upper threshold of the contrast limit. Default: (1, 4).
- tile_grid_size ((int, int)) – Grid size for histogram equalization. Default: (8, 8).

Solarize
- Invert pixel values above the threshold (solar)
- threshold ((int, int) or int, or (float, float) or float) – Inversion threshold. Default: 128.

Dropout system
Cutout
- Rough Dropout in rectangular area
- num_holes (int) – Number of regions to drop to zero. Defalt: 8.
- max_h_size (int) – Maximum height of the area. Defalt: 8.
- max_w_size (int) – Maximum width of the area. Defalt: 8.
- fill_value (int, float, lisf of int, list of float). Pixel value of the dropped area. Defalt: 0.

CoarseDropout
- Rough dropout of rectangular area (minimum value can be specified)
- max_holes (int) – Maximum number of regions to drop to zero.
- max_height (int) – Maximum height of the area. Defalt: 8.
- max_width (int) – Maximum width of the area. Defalt: 8.
- min_holes (int) – The minimum number of regions to drop to zero. Default: None.
- min_height (int) – Minimum height of the area. Default: None.
- min_width – The minimum width of the area. Default: None.
- fill_value (int, float, lisf of int, list of float). Pixel value of the dropped area. Defalt: 0.

Weather change / environment / optical reproduction system
RandomSnow
- Simulate snow
- snow_point_lower (float) – The lower limit for snow. Default: 0.1.
- snow_point_upper (float) – Upper limit for snow. Default: 0.3.
- brightness_coeff (float) – Higher values result in more snow. Should be> = 0. Default: 2.5.

RandomRain
- Rain effect
- slant_lower – Diagonal down condition. Default: -10.
- slant_upper – Diagonal up. Default: 10.
- drop_length – The length of the rain. Default: 20.
- drop_width – The width of the rain. Default: 1.
- drop_color (list of (r, g, b)) – The color of the rain line. Default: (200, 200, 200).
- blur_value (int) – A raindrop blur. Default: 7.
- brightness_coefficient (float) – Brightness. Default: 0.7.
- rain_type – The type of rain. [None, “drizzle”, “heavy”, “torrestial”]. Default: None.

RandomFog
- Simulate fog
- fog_coef_lower (float) – Lower limit of fog intensity.
- fog_coef_upper (float) – Upper limit of fog intensity.

RandomSunFlare
- Simulate solar flares
- flare_roi (float, float, float, float) – The area where flares appear (x_min, y_min, x_max, y_max).
- angle_lower (float) – Lower limit of angle.
- angle_upper (float) – Upper limit of angle.
- num_flare_circles_lower (int) – Lower limit for the number of flares.
- num_flare_circles_upper (int) – Maximum number of flares.
- src_radius (int) – Flare radius.
- src_color ((int, int, int)) – Flare color.

RandomShadow
- Simulate shadows
- shadow_roi (float, float, float, float) – The area where the shadow appears (x_min, y_min, x_max, y_max).
- num_shadows_lower (int) – Lower limit for the number of shadows.
- num_shadows_upper (int) – Maximum number of shadows.
- shadow_dimension (int) – The number of sides of the shadow polygon.

other
FancyPCA
- Extension with Fancy PCA
- alpha (float) – How much to scale the eigenvectors and eigenvalues.

PadIfNeeded
- Pad the edges of the image for the desired resolution
- min_height (int) – Minimum image height. Default: 1024.
- min_width (int) – Minimum image width. Default: 1024.
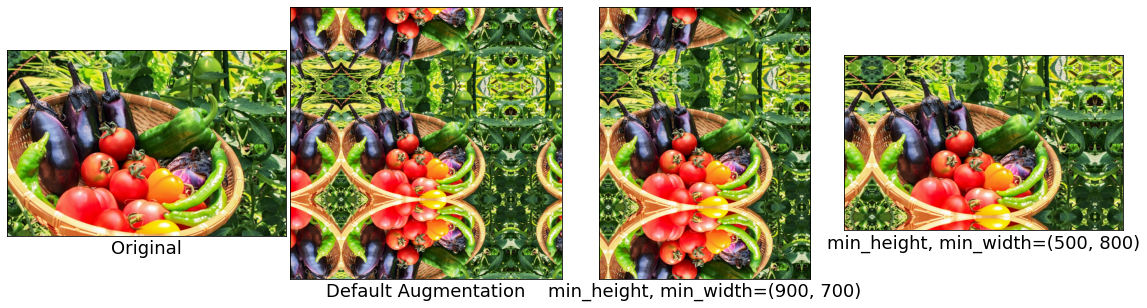