This article deepens my understanding by writing a Rails tutorial commentary article to further solidify my knowledge It is part of my study. In rare cases, ridiculous content or incorrect content may be written. Please note. I would appreciate it if you could tell me implicitly ...
Source Rails Tutorial 6th Edition
The style is not applied to the view created before, so I will arrange it with Bootstrap. You will also learn about functions around the view such as partial and Asset Pipeline.
Bootstrap published by Twitter can easily apply sophisticated designs without studying CSS very much. Make good use of Bootstrap and apply styles.
I will briefly explain the key code.
-``` [If It IE 9] `` `: Called a conditional comment. Supported by IE. This time I used it to read HTML5 shim under IE9.
-``` Link_to "link_text", "URL" `` `: One of the Rails helpers. Create a link. You can also specify a named route in the URL.
-The
-CSS class container, nav, etc. have a special meaning in Bootstrap (necessary to apply Bootstrap style).
$ curl -OL https://cdn.learnenough.com/kitten.jpg
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
0 0 0 0 0 0 0 0 --:--:-- --:--:-- --:--:-- 0
100 138k 100 138k 0 0 194k 0 --:--:-- --:--:-- --:--:-- 395k
2.mv kitten.jpg app/assets/images/kitten.jpg
Bootstrap is also super convenient in that you can easily apply styles just by specifying the class. It's too convenient to be able to handle responsive design. Normally, you have to define a style for each breakpoint for each breakpoint. Bootstrap does it for you.
SCSS is called Sass. A language that extends CSS. An easier way to write CSS, such as nesting styles.
A little explanation of how to read CSS.
body{
}
When enclosed in this way, the style for the "body" tag is applied.
.center{
}
When surrounded by words with. In this way, the style for the CSS center class is applied.
#logo{
}
If you add # like this, the style will be applied to the CSS id logo.
The basic CSS selector looks like this.
It is convenient because the entire line selected by Ctrl + / is commented out. Don't rush into studying in a Windows environment.
It becomes CSS that hides all img tags.
If the underscore_ is placed at the beginning of the file name, it is treated as a partial. Example> _header.html.erb etc. By using partials, it is convenient to make the layout easier to see and to divide it into groups for each function.
Partials are loaded in the view using the render method. Do not include underscores in the file name when calling with render.
Replace the
tag with`<% = render'layouts / head'%>`
.
Template :: Error occurs because the partial to be read by the render method has not been created.
Create a new head.html.erb and paste the contents of the head tag.
erb:head.html.erb
<head>
<title><%= full_title(yield(:title)) %></title>
<%= csrf_meta_tags %>
<%= csp_meta_tag %>
<%= stylesheet_link_tag 'application', media: 'all',
'data-turbolinks-track': 'reload' %>
<%= javascript_pack_tag 'application',
'data-turbolinks-track': 'reload' %>
<%= render 'layouts/shim' %>
</head>
You can tell how to organize assets from the manifest file. The process of grouping assets is done by a Gem called Sprockets.
*= require_tree .The description specifies that all the files in the tree should be put together in the application CSS.
Files such as Sass, CoffeeScript (an extended language of JavaScript), and ERB are converted via the preprocessor engine.
The converted ones are combined by the manifest file.
By using the asset pipeline
-Combine a large number of files into one.
-Remove unnecessary spaces and display the file in a minimized and optimized state.
Thanks to this kind of processing, you can receive benefits such as reduced loading time in the browser.
#### Stylesheet with great syntax
What you can do with Sass (SCSS)
・ Nest
You can nest and write things that would normally have to be written with a separate selector.
In other words
```css
div{
color: #eee;
}
div.container{
width: 200px;
}
The description
div{
color: #eee;
.container{
width: 200px;
}
}
It can be nested like this. Nesting too much is NG, but using moderate nesting has the effect of reducing coding effort and making the structure easier to understand.
·variable Duplicate values such as CSS color codes can be stored in variables.
value;You can define variables with.
##### Exercise
1. [Rails Tutorial 6th Edition List 5.20](https://railstutorial.jp/chapters/filling_in_the_layout?version=6.0#code-refactored_scss)
### Layout link
List of named routes that can be used for now
|Page name|URL|Named route|
|:--|:--|:--|
|Home|/|root_path|
|About|/about|about_path|
|Help|/help|help_path|
|Contact|/contact|contact_path|
[From Rails Tutorial Chapter 6](https://railstutorial.jp/chapters/filling_in_the_layout?version=6.0#table-url_mapping)
#### Contact page
I did it before I knew it in Chapter 3, so I omitted it ()
Ichiou URL is included. → https://railstutorial.jp/chapters/filling_in_the_layout?version=6.0#sec-contact_page
#### Rails root URL
You can specify the routing of / by defining the rootURL
You will be able to use helper methods such as root_path and root_url.
Both of these are methods that return a URL
root_path → Returns a relative path. Because it is root /
root_url → Returns the absolute path. Because it is root, https://www.example.com/
It doesn't matter which one you use, depending on whether it's a relative path or an absolute path.
In Rails, there is a rule that the link specification at the time of redirect uses an absolute path. (Although it works with a relative path)
*** _url method when redirecting
Otherwise, specify it with the *** _ path method.
Since the home page and help page are the basic pages of the application, I want to put the URL at the top.
Currently, it is a little troublesome to put static_pages in / static_pages / help and URL.
I will change it so that I can fly with / help.
```ruby
get '/help', to: 'static_pages#help'
get '/about', to: 'static_pages#about'
get '/contact', to: 'static_pages#contact'
By rewriting the routing in this way Named routes for help, about, contact will be set, and URLs such as / help will be linked to the routing specified by to :.
If you change the routing, the method you used in the test will become old and the test will not pass. Make some corrections.
test "should get help" do
get help_path
assert_response :success
assert_select "title", "Help | #{@base_title}"
end
test "should get about" do
get about_path
assert_response :success
assert_select "title", "About | #{@base_title}"
end
test "should get contact" do
get contact_path
assert_response :success
assert_select "title", "Contact | #{@base_title}"
end
1.2. After updating the routing
get helf_path
And rewrite the test method.
In other words, you can change the name of the named route with the as option. (Default is URL)
The route helf is associated with the help action of the static_pages controller when accessing / help. means
Now that we have defined a named route, we can use the corresponding helper methods. For the time being, I will rewrite the link part that was replaced by # with a named route.
<%= link_to "contact", 'contact_path' %>
Rewrite the links such as header and footer like this.
In the previous exercise, I forgot to return to the helf root, so I can just define it as helf_path.
Ctrl + Z is convenient when returning as before (Windows people only) By the way, there are two places where helf_path is used: static_pages_controller_test.rb and _header.html.erb. Of course, edit the defined routes.rb depending on whether it is helf or help. I will not use helf anymore, so let's go back to help.
Delete the description of ```as:'helf'`` `in routes.rb.
Change helf_path in static_pages_controller_test.rb to help_path
Change helf_path in _header.html.erb to help_path
You can also manually check each link to see if each link in the layout works properly. It's easier and more reliable to test automatically. In this case, I want to check all the links of static_pages, so I will write an integration test.
Here is the code I wrote for the integration test I added this time
test "layout links" do
get root_path
assert_template 'static_pages/home'
assert_select "a[href=?]",root_path, count: 2
assert_select "a[href=?]",help_path
assert_select "a[href=?]",about_path
assert_select "a[href=?]",contact_path
end
I send a GET request to the root path to see if I've seen the home view and see if each link exists.
Here we use assert_select a bit more advanced. Looks like If you put the link path in the second argument, it will only be assigned to the? Part. count: 2 just checks to see if there are two of those elements. A simple method if you know it.
There are many other ways to use it. Several examples of using assert_select (from Rails tutorial 6th edition)
By specifying integration, it can be executed only for integration tests, but basically it is an automatic test of guard
```rails t```Even if you run all the tests with, there is no difference in execution time so much```rails t```I think it's okay.
And here my test got an error
14:18:53 - INFO - Running: test/integration/site_layout_test.rb Running via Spring preloader in process 10766 Started with run options --seed 5553
FAIL["test_layout_links", #<Minitest::Reporters::Suite:0x000055eebbcd1e00 @name="SiteLayoutTest">, 1.4094162390028941] test_layout_links#SiteLayoutTest (1.41s) Expected exactly 2 elements matching "a[href="/"]", found 3.. Expected: 2 Actual: 3 test/integration/site_layout_test.rb:7:in `block in class:SiteLayoutTest'
1/1: [=====================================================] 100% Time: 00:00:01, Time: 00:00:01
Finished in 1.41642s 1 tests, 2 assertions, 1 failures, 0 errors, 0 skips
Apparently, the number of root URL links expected in the test code is two, but in reality there are three.
Except for the code and the actual application execution screen
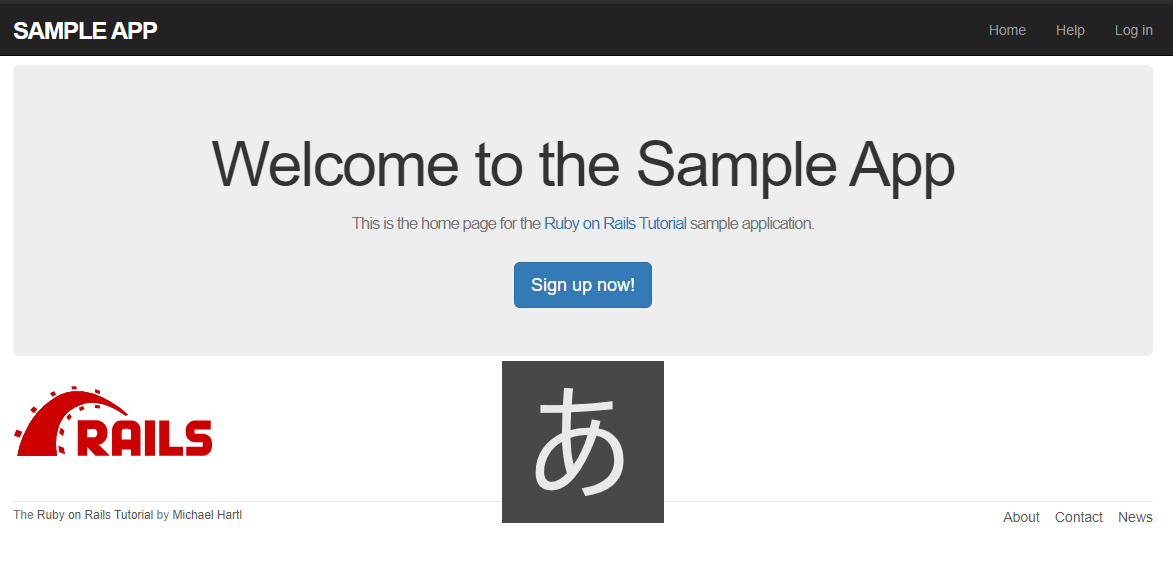
Apparently in the scss exercise I did earlier
img{ display: none; }
It seems that there are three links because I did not add it by skipping.
It's annoying, so I deleted the line with image_tag and it was cured ()
In this way, you can easily see the mistaken part in the test execution result.
Write the test as appropriate ...
##### Exercise
1. Similar to the previous example
Expected at least 1 element matching "a[href="/about"]", found 0..
Expected 0 to be >= 1.
test/integration/site_layout_test.rb:9:in `block in <class:SiteLayoutTest>'
The path to / about is 0, I'm angry that there should be more than one.
This shows that the description of about_path is missing.
2.
#### **`application_helper_test.rb`**
```rb
require 'test_helper'
class ApplicationHelperTest < ActionView::TestCase
test "full title helper" do
assert_equal full_title, "Ruby on Rails Tutorial Sample App"
assert_equal full_title("Help"), "Help | Ruby on Rails Tutorial Sample App"
end
end
Start creating a user registration page.
Create a users controller with the rails generate command as in static_pages.
$ rails g controller users new
get'/ signup', to:'users # new'
Rewrite like this.Correct the part that caused the error in the previous exercise. Specifically, make the automatically generated test code correspond to / signup routing.
users_controller_test.rb
test "should get new" do
get signup_path
assert_response :success
end
The test passes by rewriting to signup_path in this way.
After that, apply signup_path to the link on the home page Edit the auto-generated signup page.
get signup_path
assert_select "title", full_title("Sign up")
Add these two lines to site_layout_test.rb.
Commit and push this change with git as usual.
Recommended Posts