-[Latest] How to build Java environment on Ubuntu
-[Even beginners can do it! ] How to create a Java environment on Windows 10 (JDK14.0.1)
-[Easy-to-understand explanation! ] How to use Java instance
-[Even beginners can do it! ] How to install Eclipse on Windows 10 (Java environment construction)
-[Easy-to-understand explanation! ] How to use Java encapsulation
-[Easy-to-understand explanation! ] How to use Java inheritance [Override explanation]
-[Easy-to-understand explanation! ] Type conversion of reference type in Java
As prior knowledge, the contents of the above link are required.
--Polymorphism
means that objects with different processing
can be handled by the same operation
.
--The operating side
can use the operation without being aware of the difference in the
processing of the object.
--Polymorphism
is also called diversity
・ polymorphism
・ polymorphism
.
--Polymorphism
is realized by treating an instance of a subclass that inherits the abstract class
with the abstract class type
.
--A abstract class
is a class created on the assumption that it will be inherited.
--The abstract class
has afeature that cannot be instantiated. --In the
abstract class, you can define a"
abstract method" that is supposed to be
overridden. --Define
abstract class and
abstract method with "ʻabstract modifier
".
--The abstract method
cannot have the private
/ static
/ final
qualifier.
--When using the abstract class
, define a subclass
that inherits the abstract class
andoverride the
abstract method. --A class that inherits the
abstract class is called a
concrete class`.
Test class
package package name;
public class main class name{
public static void main(String[] args) {
//Instance generation
Subclass name variable name=new subclass name();
//Execution of processing
Variable name.Abstract method name(Actual argument)
}
}
Abstract class
package package name;
abstract class abstract class name{
//Definition of instance variables
private type name variable name;
//Constructor (executed when instantiating)
Abstract class name(Type name argument){
Initialization process, etc.
}
//Abstract method
abstract abstract method name(Type name argument);
}
Subclass
package package name;
class subclass name extends abstract class name{
//Definition of instance variables
private type name variable name;
//Constructor (executed when instantiating)
Subclass name(Type name argument){
super(argument);
Initialization process, etc.
}
//override(Abstract method)
@Override
Abstract method name(Type name argument){
//processing
}
}
--Basic polymorphism
is written as above.
[File (F)]-> [New (N)]-> [Java Project]
.
Test1
for the project name and click the Done
button.
[File (F)] → [New (N)] → [Class]
.
Test1
for the package and name and click the Done
button.
Test1.java
has been created.
Test1
in the package and Greeting
in the name, check ʻabstract, and click the
Finishbutton in the same procedure as in 6.3. 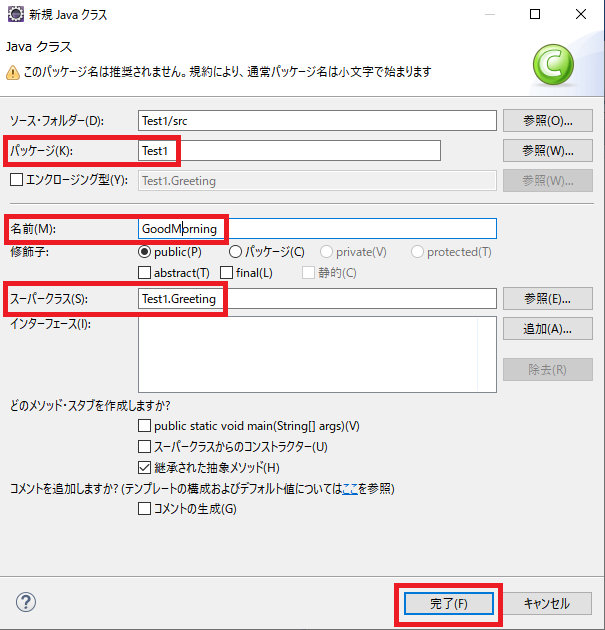 Enter
Test1 in the package,
GoodMorningin the name, and
Test1.Greeting in the superclass, as in 8.3, and click the
Finishbutton. 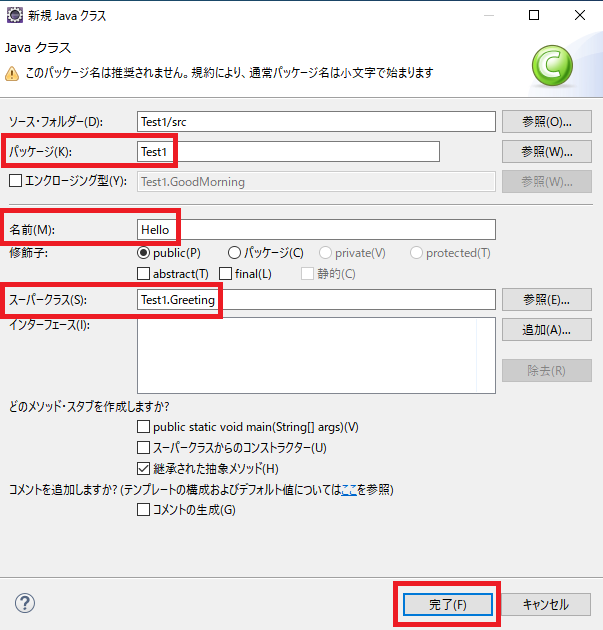 Enter
Test1 in the package,
Helloin the name, and
Test1.Greeting in the superclass, as in 9.3, and click the
Finishbutton. 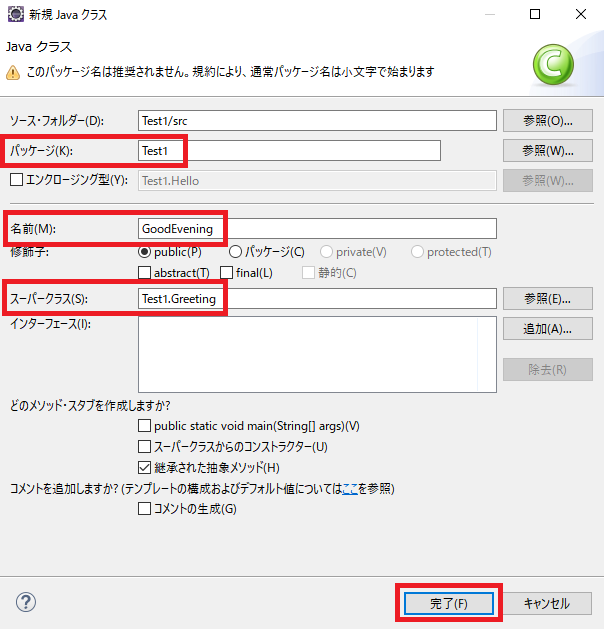 Enter
Test1 in the package,
GoodEveningin the name, and
Test1.Greeting in the superclass, as in 10.3, and click the
Finish` button.
Test1.java
, Greeting.java
, GoodMorning.java
, Hello.java
, and GoodEvening.java
are created.Test1.java
package Test1;
public class Test1 {
public static void main(String[] args) {
//Instance generation
Greeting[] greeting = {new GoodMorning("A"),new GoodMorning("B"),new GoodMorning("C")};
//Greeting display
for(int i = 0;i < greeting.length;i++) {
greeting[i].showGreeting();
}
}
}
Greeting.java
package Test1;
public abstract class Greeting {
//Instance variables
private String name;
//constructor
public Greeting(String name) {
this.name = name;
}
// getter
public String getName() {
return name;
}
//Display greetings
abstract void showGreeting();
}
Greeting.java
package Test1;
public class GoodMorning extends Greeting {
//constructor
public GoodMorning(String name) {
super(name);
}
//Display greetings
@Override
void showGreeting() {
System.out.println(getName()+"Good morning, Mr.");
}
}
--Copy the above sentence, specify S-JIS
as the character code, save the file name as Test1.java
, Greeting.java
, GoodMorning.java
, and execute it. .. ↓ ↓
--Object-oriented
treats any event
as a object
.
--Therefore, the number of classes to be instantiated
increases in proportion to thenumber of objects``. ――If you think of
writing code for the number of objects, it becomes a tremendous number and it is difficult to write it down. --
Polymorphism finds commonalities between objects and abstracts them to
simplify the code and `reduce the amount of code written``.
--A, B, C greet in order.
Good morning '","
Hello", three types of"
Good evening". --If you do not do
polymorphism` when creating a system like the one above, the amount of code will increase and it will become complicated as shown in the code below.Test1.java
package Test1;
public class Test1 {
public static void main(String[] args) {
//Instance generation
GoodMorning[] goodmorning = {new GoodMorning("A"),new GoodMorning("B"),new GoodMorning("C")};
Hello[] hello = {new Hello("A"),new Hello("B"),new Hello("C")};
GoodEvening[] goodevening = {new GoodEvening("A"),new GoodEvening("B"),new GoodEvening("C")};
//Greeting display
for(int i = 0;i < goodmorning.length;i++) {
goodmorning[i].showGreeting();
}
for(int i = 0;i < hello.length;i++) {
hello[i].showGreeting();
}
for(int i = 0;i < goodevening.length;i++) {
goodevening[i].showGreeting();
}
}
}
Hello.java
package Test1;
public class Hello {
//Instance variables
String name;
//constructor
public Hello(String name) {
this.name = name;
}
//Display greetings
void showGreeting() {
System.out.println(name+"Hey,.");
}
}
GoodMorning.java
package Test1;
public class GoodMorning{
//Instance variables
String name;
//constructor
public GoodMorning(String name) {
this.name = name;
}
//Display greetings
void showGreeting() {
System.out.println(name+"Good morning, Mr.");
}
}
GoodEvening.java
package Test1;
public class GoodEvening {
//Instance variables
String name;
//constructor
public GoodEvening(String name) {
this.name = name;
}
//Display greetings
void showGreeting() {
System.out.println(name+"Good evening, Mr.");
}
}
--Copy the above sentence, specify S-JIS
as the character code, and save the file name as Test1.java
, GoodMorning.java
, Hello.java
, GoodEvening.java
. , When executed, it becomes like this. ↓ ↓
--In the above example, polymorphism is not used
, so there arethree
arrays, and there is a corresponding
for statement. --You can combine
arrays into one by
abstracting using
polymorphism. --The following is the
abstraction of
GoodMorning.java,
Hello.java, and
GoodEvening.java` in the above example.
Test1.java
package Test1;
public class Test1 {
public static void main(String[] args) {
//Instance generation
Greeting[] greeting = {
new GoodMorning("A"),new GoodMorning("B"),new GoodMorning("C"),
new Hello("A"),new Hello("B"),new Hello("C"),
new GoodEvening("A"),new GoodEvening("B"),new GoodEvening("C")
};
//Greeting display
for(int i = 0;i < greeting.length;i++) {
greeting[i].showGreeting();
}
}
}
Greeting.java
package Test1;
public abstract class Greeting {
//Instance variables
private String name;
//constructor
public Greeting(String name) {
this.name = name;
}
// getter
public String getName() {
return name;
}
//Display greetings
abstract void showGreeting();
}
Hello.java
package Test1;
public class Hello extends Greeting{
//constructor
public Hello(String name) {
super(name);
}
//Display greetings
@Override
void showGreeting() {
System.out.println(getName()+"Hey,.");
}
}
GoodMorning.java
package Test1;
public class GoodMorning extends Greeting {
//constructor
public GoodMorning(String name) {
super(name);
}
//Display greetings
@Override
void showGreeting() {
System.out.println(getName()+"Good morning, Mr.");
}
}
GoodEvening.java
package Test1;
public class GoodEvening extends Greeting {
//constructor
public GoodEvening(String name) {
super(name);
}
//Display greetings
@Override
void showGreeting() {
System.out.println(getName()+"Good evening, Mr.");
}
}
--Copy the above sentence, specify S-JIS
as the character code, and name the file``Test1.java,
Greeting.java,
GoodMorning.java,
Hello.java,
GoodEvening. If you save it as .java` and run it, it will look like this. ↓ ↓
-[Useful to remember !!!] Easy creation of constructor and getter / setter in Eclipse -[Useful to remember !!!] Easy creation of inherited class in Eclipse -[Even beginners can do it! ] How to write Javadoc -[Easy-to-understand explanation! ] How to use Java overload
Recommended Posts