[In-house study session] Introduction of "Readable Code"
0. Introduction
Target person
- Programmer (C ++, Python, Java, JavaScript)
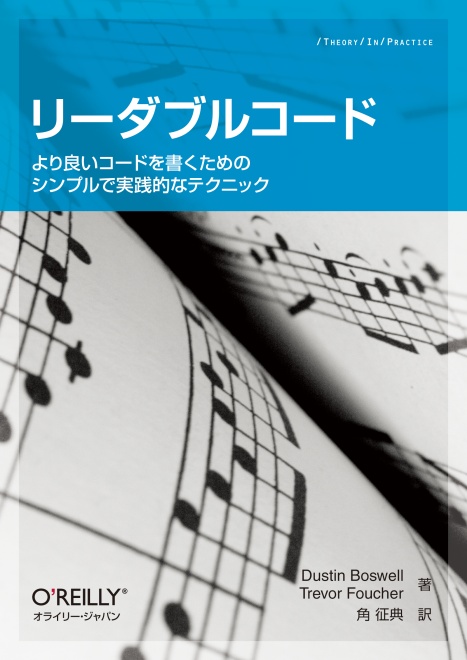
What i want to tell
- Pick up examples from the following viewpoints from "Readable Code"
--Items often pointed out in the review
--Items that are easy to explain
- Please use this as an opportunity to read the "Readable Code". Books are more systematic and easier to understand. Everything written is important.
Notes
- Basically, only the title is posted, and the sample code is not posted. You need to read a book to fully understand it.
- The content written as [Supplement] is not listed in the "Readable Code".
Table of contents of "Readable Code"
Chapter 1 Easy-to-understand code
Part I Superficial improvements
Chapter 2 Packing information in the name
Chapter 3 Names that are not misunderstood
Chapter 4 Beauty
Chapter 5 Know what to comment
Chapter 6 Comments are accurate and concise
Part II: Simplification of loops and logic
Chapter 7 Make the control flow easier to read
Chapter 8 Divide a huge formula
Chapter 9 Variables and readability
- Chapter 10 and beyond will not be introduced, so omitted
Introduction of "Readable Code"
Chapter 1 Easy-to-understand code
-
The code should be easy to understand.
--The most important principle in writing code
-
The code should be written so that others can understand it in the shortest possible time.
-"Basic theorem of readability"
-
"Time to understand" does not compete with other goals at all
--Easy-to-understand code often leads to good design and ease of testing.
Chapter 2 Packing information in the name
2.2 Avoid generic names such as tmp and retval
- There is no information in the name retval. Use a name that represents the value of the variable.
Use the name * tmp only for variables that have a short lifetime and where temporary completion is most important.
2.5 Determine the length of the name
- Short names are fine as long as the scope is small
2.6 Communicate information in name format
-
You can stuff names with information using underscores, dashes, and capital letters.
-
[Supplement] See below for Java format
Chapter 3 Names that are not misunderstood
- Ask yourself many times that the name is "Isn't it mistaken for another meaning?"
- Use min and max to indicate limits
- Use first and last to indicate range
- Use begin and end for inclusive / exclusive ranges
Supplement
- Note the antonyms. The antonym of start is stop instead of end.
http://webspace.jugem.jp/?eid=947
3.6 Boolean name
The meaning of true and false must be clarified.
The following variables can be interpreted in two ways.
bool read_password = true;
- Password needs to be read ** from now on **.
- Password has been ** already ** read
It is better to use need_password
or ʻuser_is_authenticate`.
- Boolean variable names are often prefixed with ʻis, has, can, should`, etc. to make them easier to understand.
Chapter 4 Beauty
Three principles
- Use a layout that is consistent with the patterns your readers are accustomed to.
- Similar code makes it look similar
- Block related code together.
Chapter 5 Know what to comment
5.2 What should not be commented
- Don't comment on what you can tell from the code
- Rename terrible names without commenting
-"Good code> Terrible code + Good comment"
5.3 Record your thoughts
- Why the code looks like this rather than the other way around ("Director's Commentary")
- "Background" related to constant values
[Personal view]
It seems better to write the background not only in the source but also in the table name and column name of the database.
7.5 Return quickly from function
Some people think that functions should not use multiple return
minutes. Stupid. It's good to return quickly from the function. Rather, it is sometimes desirable.
~
The reason I want to have one function exit is because I want to make sure that some cleanup code is executed. Modern languages offer this mechanism in a more sophisticated way.
In Java, try ... finally
is an idiom for cleanup code.
[Supplement] The source of "return prohibition other than the end of the function"
MISRA-C, a "software design standard for C language", seems to be the source.
http://d.hatena.ne.jp/eel3/20121225/1356443485
9.2 Reduce the scope of variables
- Reduce the number of lines of code where variables are visible as much as possible.
- Lower the definition position.
--Variable definition moves just before using variable
--In the original C language, it was necessary to define variables at the beginning of functions and blocks.
appendix
Java general naming rules
- Class name: Camel case starting with a capital letter
- Variable: Camel case starting with a lowercase letter
- Constant: All uppercase, snake case (underscore delimited)
- Package: All lowercase
- Name variables with nouns and methods with verbs
Reference coding standards
Personal view of coding conventions
-
Do not bring in circumstances in other languages
--Do not bring C language rules into Java
――Including indent style etc.
-
Be aware of the times
--With the development of IDE, the definition of easy-to-read code changes a little.
--Java used to put an underscore at the end of a field. It is not recommended because it is displayed in different colors in the IDE now.
https://www.acroquest.co.jp/webworkshop/javacordingrule/Acroquest_JavaCodingStandard_7_0.pdf
――The contents of the "readable code" may eventually become old-fashioned.
Reference site
http://qiita.com/AKB428/items/20e81ccc8d9998b5535d